Table of Contents
HTML Code for Website
Creating a website from scratch can seem like a daunting task, but understanding HTML (HyperText Markup Language) is the first step to demystifying web development. HTML forms the backbone of any website, defining its structure and content. This article will guide you through the essentials of HTML, offering a comprehensive look at how to write HTML code for a website, including practical examples and best practices.
Outline HTML Code for Website
- Introduction HTML Code for Website
- Importance of HTML in Web Development
- Overview of HTML Structure
- Getting Started with HTML
- Basic HTML Document Structure
- HTML Tags and Attributes
- Creating a Simple HTML Page
- Doctype Declaration
- HTML, Head, and Body Tags
- Adding Content to Your HTML Page
- Headings
- Paragraphs
- Lists (Ordered and Unordered)
- Formatting Text
- Bold, Italic, and Underline
- Creating Line Breaks and Horizontal Lines
- Adding Links and Images
- Anchor Tags
- Image Tags
- Creating Tables
- Basic Table Structure
- Adding Rows and Columns
- Forms and Input Elements
- Creating a Simple Form
- Different Input Types
- Using HTML5 Elements
- Semantic Elements (header, footer, article, section)
- Multimedia Elements (audio, video)
- CSS Integration
- Inline CSS
- Internal CSS
- External CSS
- Best Practices in HTML Coding
- Proper Nesting
- Closing Tags
- Commenting Code
- Debugging HTML Code
- Common HTML Errors
- Tools for Debugging
- SEO and HTML
- Importance of Semantic HTML
- Meta Tags
- Advanced HTML Features
- HTML APIs
- HTML with JavaScript
- Conclusion
- Recap of Key Points
- Encouragement to Practice
- FAQs
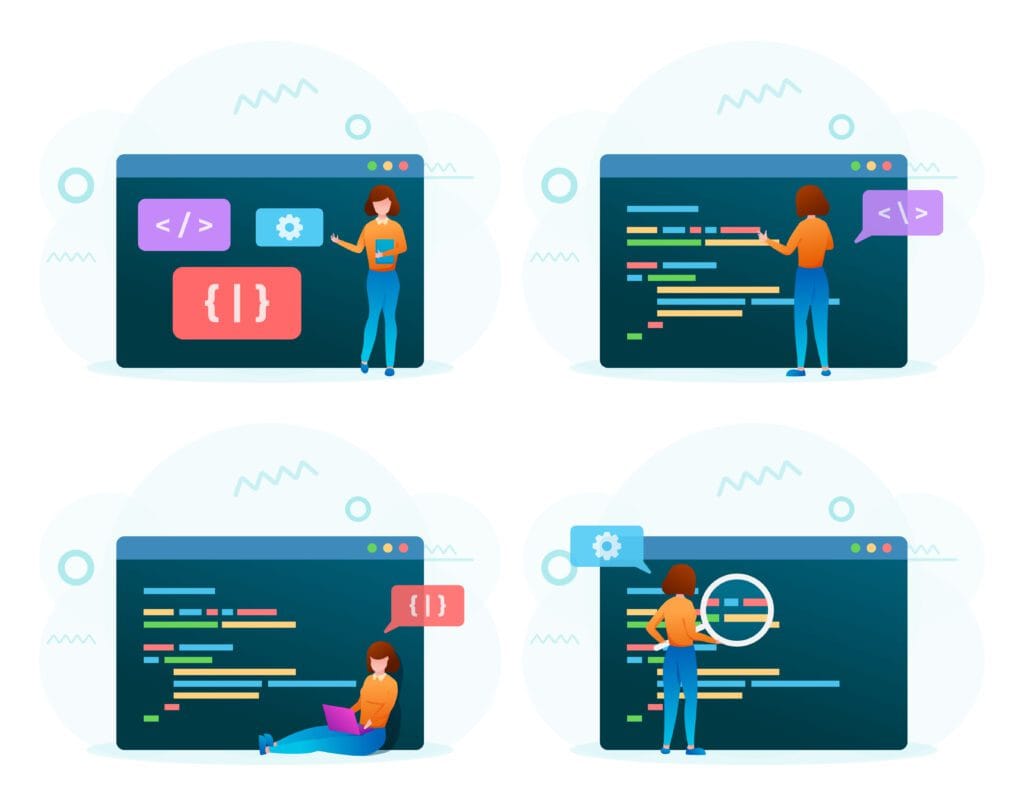
Introduction HTML Code for Website
Importance of HTML in Web Development
HTML is the foundational language of the web, providing the essential structure for web pages. Without HTML, the internet as we know it would not exist. It enables web browsers to display text, images, links, and other media in a structured and visually appealing way.
Overview of HTML Structure
At its core, HTML consists of a series of elements, represented by tags, which define different parts of a web page. These elements include headings, paragraphs, links, images, tables, and forms, among others. Understanding how to use these elements effectively is crucial for creating well-structured and accessible websites.
Getting Started with HTML
Basic HTML Document Structure
Every HTML document starts with a declaration and contains a head and a body. The head includes metadata and links to external resources, while the body contains the content that is displayed on the web page.
HTML Tags and Attributes
HTML tags are the building blocks of HTML. They are enclosed in angle brackets and usually come in pairs, with an opening tag and a closing tag. Attributes provide additional information about elements and are included within the opening tag.
Creating a Simple HTML Page
Doctype Declaration
The first line of any HTML document is the doctype declaration, which informs the web browser about the version of HTML being used. For HTML5, it is simply:
<!DOCTYPE html>
HTML, Head, and Body Tags
The main structure of an HTML document looks like this:
<!DOCTYPE html>
<html>
<head>
<title>My First HTML Page</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<p>This is my first paragraph.</p>
</body>
</html>
Adding Content to Your HTML Page
Headings
Headings are used to create a hierarchical structure in your content, with <h1>
being the highest level and <h6>
the lowest.
<h1>Main Heading</h1>
<h2>Subheading</h2>
<h3>Sub-subheading</h3>
Paragraphs
Paragraphs are created using the <p>
tag and are used for blocks of text.
<p>This is a paragraph of text.</p>
Lists (Ordered and Unordered)
Lists can be ordered (numbered) or unordered (bulleted).
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
<ol>
<li>First item</li>
<li>Second item</li>
</ol>
Formatting Text
Bold, Italic, and Underline
Text can be emphasized using <b>
, <i>
, and <u>
tags for bold, italic, and underline, respectively.
<p>This is <b>bold</b> text.</p>
<p>This is <i>italic</i> text.</p>
<p>This is <u>underlined</u> text.</p>
Creating Line Breaks and Horizontal Lines
Line breaks and horizontal lines are created using <br>
and <hr>
tags.
<p>First line.<br>Second line.</p>
<hr>
<p>New section after horizontal line.</p>
Adding Links and Images
Anchor Tags
Links are created using the <a>
tag with the href
attribute.
<a href="https://www.example.com">Visit Example.com</a>
Image Tags
Images are added using the <img>
tag with src
and alt
attributes.
<img src="image.jpg" alt="Description of image">
Creating Tables
Basic Table Structure
Tables are created using the <table>
, <tr>
, <th>
, and <td>
tags.
<table>
<tr>
<th>Header 1</th>
<th>Header 2</th>
</tr>
<tr>
<td>Data 1</td>
<td>Data 2</td>
</tr>
</table>
Adding Rows and Columns
Additional rows and columns can be added by including more <tr>
and <td>
tags.
Forms and Input Elements
Creating a Simple Form
Forms are created using the <form>
tag with various input elements inside.
<form action="/submit" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Submit">
</form>
Different Input Types
HTML supports various input types such as text, password, email, and submit.
<input type="email" id="email" name="email">
<input type="password" id="password" name="password">
Using HTML5 Elements
Semantic Elements (header, footer, article, section)
HTML5 introduced new semantic elements that provide better structure and meaning.
<header>
<h1>Website Header</h1>
</header>
<section>
<h2>Section Title</h2>
<p>Content of the section.</p>
</section>
<footer>
<p>Website Footer</p>
</footer>
Multimedia Elements (audio, video)
HTML5 also supports multimedia elements for embedding audio and video.
<video controls>
<source src="video.mp4" type="video/mp4">
Your browser does not support the video tag.
</video>
<audio controls>
<source src="audio.mp3" type="audio/mp3">
Your browser does not support the audio element.
</audio>
CSS Integration
Inline CSS
CSS can be added directly within HTML tags using the style
attribute.
<p style="color:blue;">This is a blue paragraph.</p>
Internal CSS
CSS can be included within the <style>
tag in the head section.
<head>
<style>
p { color: red; }
</style>
</head>
External CSS
CSS can be linked from an external stylesheet using the <link>
tag.
<head>
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
Best Practices in HTML Coding
Proper Nesting
Ensure tags are properly nested to avoid rendering issues.
<p>This is <b>properly nested</b> tags.</p>
Closing Tags
Always close tags to maintain valid HTML.
<p>This is a paragraph.</p>
Commenting Code
Use comments to explain sections of your code.
<!-- This is a comment -->
Debugging HTML Code
Common HTML Errors
Look out for common errors like unclosed tags and incorrect nesting.
Tools for Debugging
Use browser developer tools and online validators like the W3C validator.
SEO and HTML
Importance of Semantic HTML
Using semantic HTML
improves accessibility and SEO.
Meta Tags
Meta tags provide metadata about the HTML document.
<head>
<meta name="description" content="Description of the webpage">
</head>
Advanced HTML Features
HTML APIs
HTML5 includes APIs for advanced functionalities like geolocation and local storage.
HTML with JavaScript
Combine HTML with JavaScript to create dynamic and interactive web pages.
<script>
document.getElementById("demo").innerHTML = "Hello JavaScript!";
</script>
Conclusion
Understanding HTML is fundamental to web development. By mastering the basics outlined in this article, you can create well-structured, functional, and visually appealing websites. Remember to follow best practices and continuously practice coding to improve your skills.
FAQs
- What is HTML?
HTML (HyperText Markup Language) is the standard language for creating web pages and web applications. - Why is HTML important for web development?
HTML provides the structure of a web page, allowing browsers to display content correctly. - Can I create a website using only HTML?
Yes, but for a fully functional and styled website, you’ll need to use CSS and JavaScript as well. - What are HTML tags?
HTML tags are the building blocks of HTML, used to define elements within a web page. - How can I learn HTML?
Practice coding, use online resources, and follow tutorials to learn and improve your HTML skills.
Wow! After all I got a weblog from where I can actually take valuable information regarding my study and knowledge.
Multimedia Engineering
Informatics Engineering
Internet Engineering
Language Center
International Studies
Electronics Engineering
telecoms
electrical engineering
computer engineering
It’s a shame you don’t have a donate button! I’d
certainly donate to this superb blog! I suppose for now
i’ll settle for bookmarking and adding your RSS feed to
my Google account. I look forward to fresh updates and will talk about this website with my
Facebook group. Chat soon!