Introduction to JavaScript Basics for Beginners
What is JavaScript?
JavaScript is a versatile, high-level programming language that is essential for creating interactive and dynamic web pages. Initially developed by Netscape in 1995, it has evolved significantly and is now a core technology alongside HTML and CSS in web development.
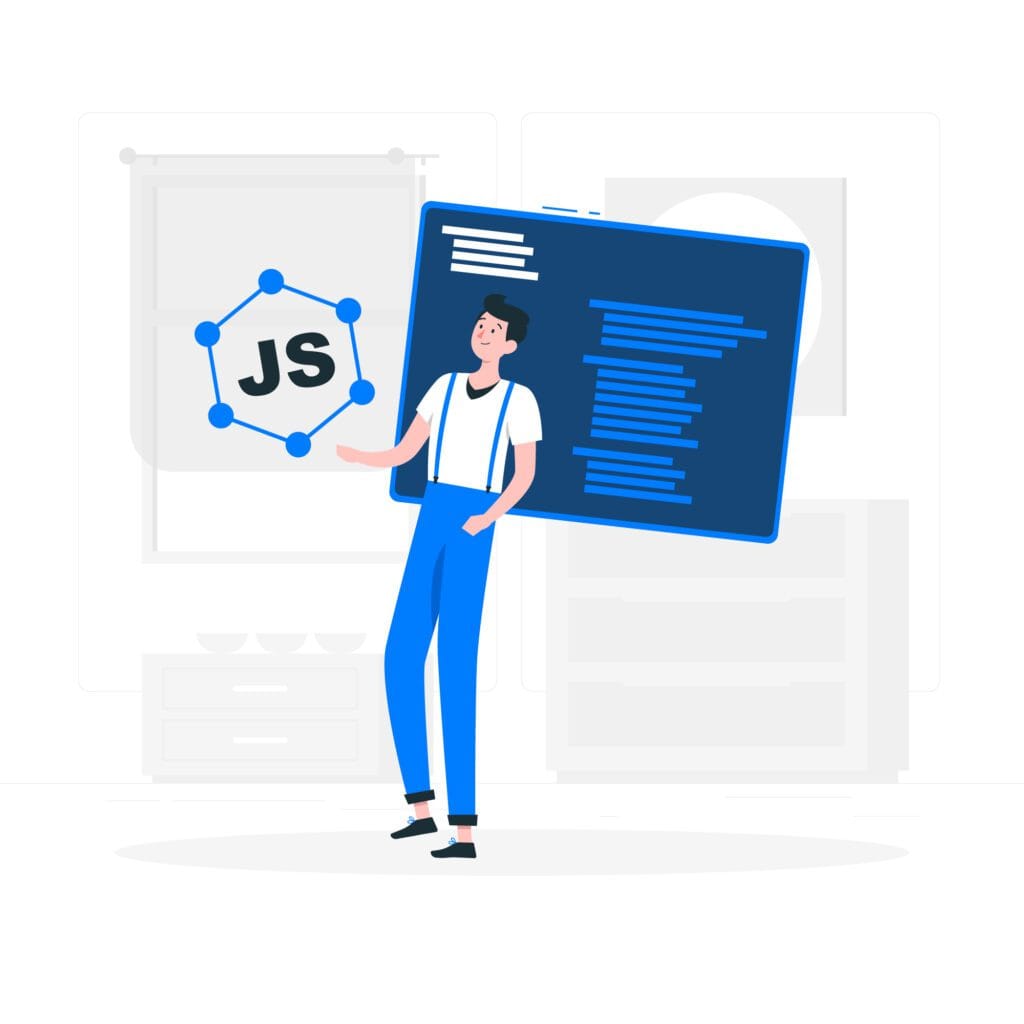
Table of Contents
History of JavaScript
JavaScript was created by Brendan Eich while working at Netscape. Originally called Mocha, it was later renamed LiveScript, and finally JavaScript. The language was standardized by the European Computer Manufacturers Association (ECMA) in 1997, leading to the development of the ECMAScript specification, which continues to guide JavaScript’s evolution.
Why Learn JavaScript?
JavaScript is ubiquitous in modern web development. Its ability to manipulate the DOM, handle events, and interact with servers makes it indispensable. Learning JavaScript opens doors to careers in front-end, back-end, and even mobile app development, thanks to its versatility and the vast ecosystem of libraries and frameworks.
JavaScript in Modern Web Development
Today, JavaScript is not just limited to client-side scripting. With the advent of Node.js, it can also be used for server-side development, making it possible to build entire web applications using JavaScript alone. Additionally, frameworks like React, Angular, and Vue have revolutionized front-end development, enabling the creation of complex, scalable, and maintainable user interfaces.
Setting Up Your Development Environment
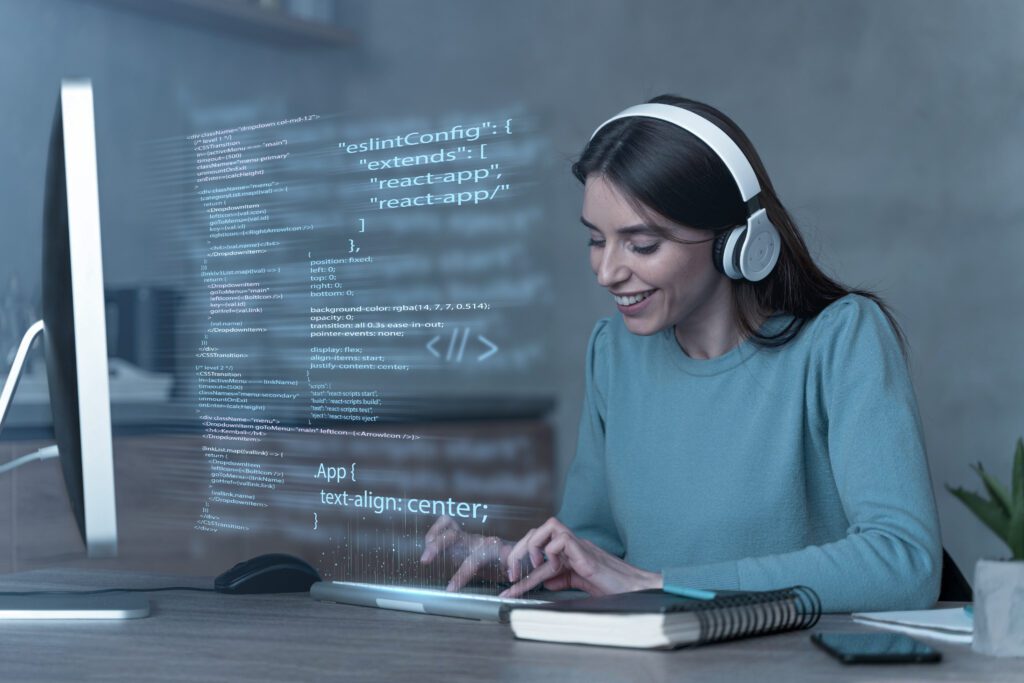
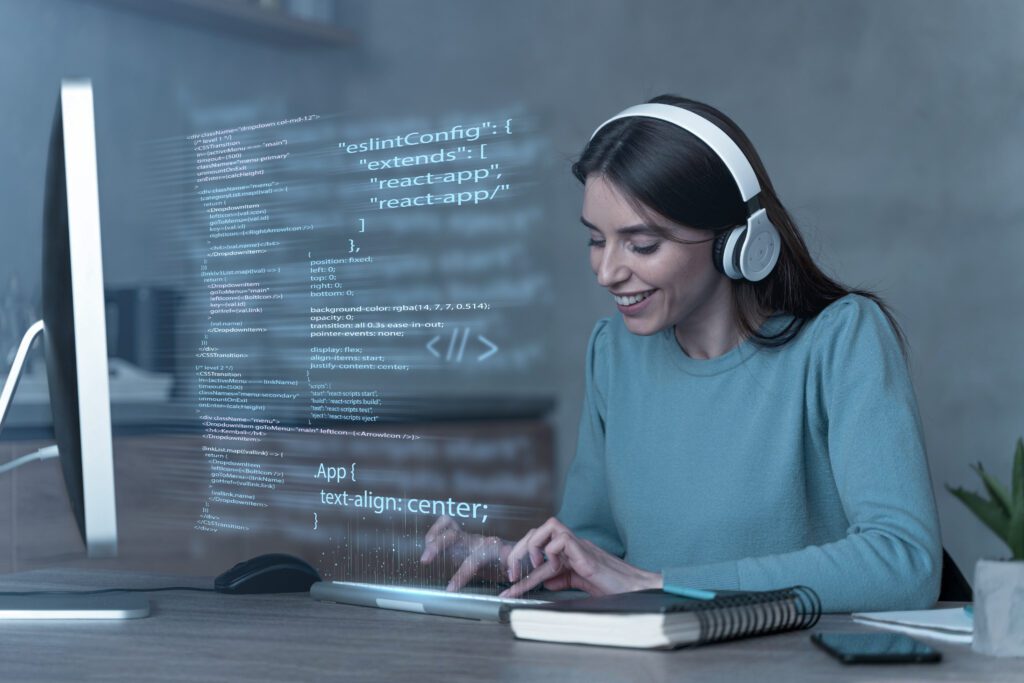
Choosing a Text Editor
A good text editor is crucial for writing efficient and error-free JavaScript code. Popular choices include Visual Studio Code, Sublime Text, and Atom. These editors offer features like syntax highlighting, code snippets, and integrated debugging tools that enhance the coding experience.
Setting Up Node.js
Node.js is a runtime environment that allows you to run JavaScript on the server. To set it up, download and install it from the official Node.js website. Node.js comes with npm (Node Package Manager), which is used to install libraries and frameworks, making it a valuable tool for JavaScript developers.
Using the Browser Console
The browser console is a powerful tool for testing and debugging JavaScript code. Most modern browsers, such as Chrome and Firefox, come with built-in developer tools that include a console. You can access the console by right-clicking on a webpage, selecting “Inspect,” and navigating to the “Console” tab.
Read More: Master Advanced HTML and CSS for Stunning Websites
JavaScript Syntax and Basics
Hello, World! Program
The “Hello, World!” program is a simple way to start learning any programming language. In JavaScript, you can write this program by using the console.log
function:
console.log("Hello, World!");
Variables and Data Types
JavaScript has several data types, including strings, numbers, booleans, objects, and arrays. Variables can be declared using var
, let
, or const
. The choice of declaration affects the scope and reusability of the variable.
let name = "John Doe";
const age = 30;
var isStudent = true;
Operators
JavaScript supports various operators, including arithmetic, comparison, logical, and assignment operators. These operators are used to perform operations on variables and values.
let sum = 10 + 20; // Arithmetic
let isEqual = (sum === 30); // Comparison
let isAdult = (age >= 18); // Logical
name += " Jr."; // Assignment
Control Structures
Control structures like if-else
, for
, while
, and switch
are used to control the flow of the program based on certain conditions.
if (isAdult) {
console.log("You are an adult.");
} else {
console.log("You are not an adult.");
}
for (let i = 0; i < 5; i++) {
console.log(i);
}
Functions in JavaScript
Defining Functions
Functions are blocks of code designed to perform a particular task. A function is executed when it is invoked or called.
function greet(name) {
return `Hello, ${name}!`;
}
console.log(greet("Alice"));
Function Expressions
Functions can also be defined as expressions and stored in variables. These are called function expressions.
const greet = function(name) {
return `Hello, ${name}!`;
};
console.log(greet("Bob"));
Arrow Functions
Arrow functions provide a more concise syntax for writing function expressions and do not have their own this
context.
const greet = (name) => `Hello, ${name}!`;
console.log(g
reet("Charlie"));
Function Scope
JavaScript has two types of scope: global and local. Variables declared inside a function are in local scope and cannot be accessed outside the function.
function scopeTest() {
let localVariable = "I'm local";
console.log(localVariable);
}
scopeTest();
// console.log(localVariable); // This will cause an error
Objects and Arrays
Introduction to Objects
Objects are collections of key-value pairs. They are used to store data in a structured way.
let person = {
name: "John Doe",
age: 30,
isStudent: true
};
console.log(person.name);
Object Methods
Objects can also contain functions, which are called methods. Methods are used to perform actions on the object’s data.
let person = {
name: "John Doe",
age: 30,
greet: function() {
return `Hello, my name is ${this.name}`;
}
};
console.log(person.greet());
Arrays and Array Methods
Arrays are ordered collections of values. JavaScript provides many methods to manipulate arrays, such as push
, pop
, shift
, unshift
, and splice
.
let numbers = [1, 2, 3, 4, 5];
numbers.push(6); // Adds 6 to the end
numbers.pop(); // Removes the last element
console.log(numbers);
DOM Manipulation
What is the DOM?
The Document Object Model (DOM) is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content.
Selecting Elements
JavaScript provides several methods to select elements from the DOM, such as getElementById
, getElementsByClassName
, getElementsByTagName
, querySelector
, and querySelectorAll
.
let element = document.getElementById("myElement");
Modifying the DOM
Once you have selected an element, you can modify it. You can change its content, style, attributes, and more.
let element = document.getElementById("myElement");
element.textContent = "New content";
element.style.color = "red";
Event Handling
Events are actions that happen in the browser, such as clicks, keypresses, and mouse movements. JavaScript can react to these events using event listeners.
let button = document.getElementById("myButton");
button.addEventListener("click", function() {
alert("Button was clicked!");
});
JavaScript Events
Introduction to Events
Events are an integral part of JavaScript. They allow developers to make web pages interactive by responding to user actions.
Common Events
Some common events include click
, dblclick
, mouseover
, mouseout
, keydown
, keyup
, load
, and resize
.
window.addEventListener("load", function() {
console.log("Page is fully loaded");
});
Event Listeners
Event listeners are used to run a function when an event occurs. They can be added to any HTML element.
let input = document.getElementById("myInput");
input.addEventListener("keyup", function(event) {
console.log(`Key pressed: ${event.key}`);
});
Asynchronous JavaScript
Callbacks
A callback is a function passed into another function as an argument, which is then invoked inside the outer function to complete some kind of routine or action.
function fetchData(callback) {
setTimeout(() => {
callback("Data fetched");
}, 1000);
}
fetchData((message) => {
console.log(message);
});
Promises
Promises provide a cleaner way to handle asynchronous operations. They represent a value that may be available now, in the future, or never.
let promise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Promise resolved");
}, 1000);
});
promise.then((message) => {
console.log(message);
});
Async/Await
async
and await
make it easier to work with promises. async
functions return a promise, and await
pauses the function execution until the promise settles.
async function fetchData() {
let data = await new Promise((resolve) => {
setTimeout(() => {
resolve("Data fetched");
}, 1000);
});
console.log(data);
}
fetchData();
JavaScript Best Practices
Writing Clean Code
Writing clean and maintainable code is essential for any developer. This includes using meaningful variable names, keeping functions small and focused, and commenting your code where necessary.
Debugging Techniques
Debugging is an essential skill for developers. Modern browsers offer powerful debugging tools. Using console.log
statements and breakpoints can help track down and fix bugs.
let x = 10;
console.log(x); // Debugging statement
Performance Optimization
Optimizing JavaScript performance is crucial for a smooth user experience. This includes minimizing DOM access, using efficient algorithms, and leveraging browser caching.
// Example of minimizing DOM access
let listItems = document.querySelectorAll("li");
for (let i = 0; i < listItems.length; i++) {
listItems[i].style.color = "blue";
}
Advanced JavaScript Concepts
Closures
A closure is a function that retains access to its lexical scope, even when the function is executed outside that scope.
function makeCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
let counter = makeCounter();
console.log(counter()); // 1
console.log(counter()); // 2
Prototype and Inheritance
JavaScript uses prototypal inheritance, where objects inherit properties and methods from other objects.
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.greet = function() {
return `Hello, my name is ${this.name}`;
};
let john = new Person("John Doe", 30);
console.log(john.greet());
Modules
Modules allow you to break your code into separate files and use import
and export
statements to share code between files.
// utils.js
export function add(a, b) {
return a + b;
}
// main.js
import { add } from './utils.js';
console.log(add(2, 3)); // 5
JavaScript Frameworks and Libraries
Introduction to Frameworks and Libraries
JavaScript frameworks and libraries simplify the development process by providing pre-written code for common tasks. Popular options include React, Vue, and Angular.
React.js
React is a JavaScript library for building user interfaces, maintained by Facebook. It allows developers to create reusable UI components.
import React from 'react';
import ReactDOM from 'react-dom';
function App() {
return <h1>Hello, world!</h1>;
}
ReactDOM.render(<App />, document.getElementById('root'));
Vue.js
Vue is a progressive JavaScript framework for building user interfaces. It is designed to be incrementally adoptable.
import Vue from 'vue';
new Vue({
el: '#app',
data: {
message: 'Hello, Vue!'
}
});
Angular
Angular is a platform for building mobile and desktop web applications. It is maintained by Google and provides a comprehensive framework for building dynamic web apps.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `<h1>{{title}}</h1>`
})
export class AppComponent {
title = 'Hello, Angular!';
}
Testing JavaScript Code
Unit Testing
Unit testing involves testing individual components of the code to ensure they work as expected. Popular unit testing frameworks include Jest and Mocha.
// Example using Jest
test('adds 1 + 2 to equal 3', () => {
expect(1 + 2).toBe(3);
});
Integration Testing
Integration testing involves testing the combination of components to ensure they work together. Tools like Cypress and Selenium are commonly used for integration testing.
// Example using Cypress
describe('My First Test', () => {
it('Does not do much!', () => {
expect(true).to.equal(true);
});
});
Popular Testing Libraries
There are many libraries available for testing JavaScript code, each with its own strengths. Some popular ones include Jest, Mocha, Chai, and Jasmine.
JavaScript and Web APIs
Introduction to Web APIs
Web APIs are interfaces provided by browsers that allow you to interact with various aspects of the web. Common APIs include the Fetch API, Web Storage API, and Geolocation API.
Working with Fetch API
The Fetch API allows you to make network requests similar to XMLHttpRequest
. It is more powerful and flexible, using promises to handle asynchronous operations.
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Using Web Storage API
The Web Storage API provides mechanisms for storing key-value pairs in the browser. It includes localStorage
and sessionStorage
.
// Save data to localStorage
localStorage.setItem('name', 'John Doe');
// Retrieve data from localStorage
let name = localStorage.getItem('
name');
console.log(name);
JavaScript in Backend Development
Introduction to Node.js
Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. It allows you to run JavaScript on the server, enabling full-stack JavaScript development.
Building a Simple Server
Using Node.js, you can build a simple web server with just a few lines of code.
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\n');
});
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
Connecting to Databases
Node.js can be used to connect to various databases like MongoDB, MySQL, and PostgreSQL. Using libraries like mongoose
for MongoDB or sequelize
for SQL databases makes this process easier.
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true });
const db = mongoose.connection;
db.on('error', console.error.bind(console, 'connection error:'));
db.once('open', () => {
console.log('Connected to the database');
});
JavaScript in Mobile App Development
React Native
React Native allows you to build mobile apps using JavaScript and React. It lets you create truly native apps for iOS and Android.
import React from 'react';
import { Text, View } from 'react-native';
export default function App() {
return (
<View>
<Text>Hello, React Native!</Text>
</View>
);
}
Ionic
Ionic is a framework for building hybrid mobile apps using web technologies like HTML, CSS, and JavaScript.
import { Component } from '@angular/core';
import { Platform } from '@ionic/angular';
@Component({
selector: 'app-root',
templateUrl: 'app.component.html',
})
export class AppComponent {
constructor(private platform: Platform) {
this.initializeApp();
}
initializeApp() {
this.platform.ready().then(() => {
console.log('Platform ready');
});
}
}
NativeScript
NativeScript allows you to build native mobile apps using JavaScript, TypeScript, or Angular. It provides direct access to native APIs.
import { Component } from "@angular/core";
@Component({
selector: "ns-app",
templateUrl: "app.component.html",
})
export class AppComponent {
// Your TypeScript logic
}
Conclusion
Recap of Key Points
JavaScript is a powerful and versatile language that is crucial for modern web development. From basic syntax to advanced concepts, understanding JavaScript opens up numerous opportunities in front-end, back-end, and mobile app development.
Next Steps for Learners
For beginners, the next steps include practicing coding regularly, building small projects, and exploring JavaScript frameworks and libraries. Resources like online courses, tutorials, and documentation can further enhance your learning journey. Keep experimenting, keep learning, and soon you’ll be proficient in JavaScript development.