Introduction to Advanced HTML and CSS
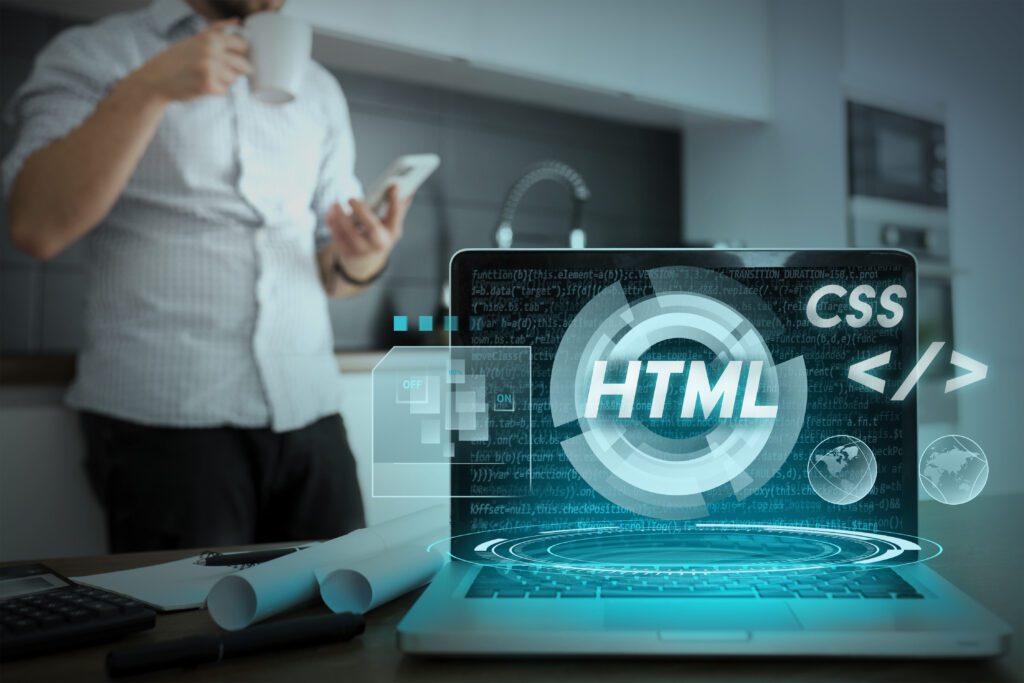
Table of Contents
What is HTML?
HTML (HyperText Markup Language) is the standard language for creating web pages and web applications. It is the foundation of any website and defines the structure of the content on the web.
What is CSS?
CSS (Cascading Style Sheets) is a style sheet language used for describing the presentation of a document written in HTML. It controls the layout of multiple web pages all at once.
Importance of Advanced HTML and CSS
Mastering advanced HTML and CSS techniques allows developers to create more complex and visually appealing websites, ensuring better user experience and accessibility.
Prerequisites for Learning Advanced HTML and CSS
Before diving into advanced topics, it’s essential to have a solid understanding of basic HTML and CSS, including knowledge of tags, attributes, basic styling, and layout techniques.
Advanced HTML Techniques
Semantic HTML
Semantic HTML refers to the use of HTML tags that convey the meaning and structure of the content. Using semantic tags improves accessibility and SEO.
<article>
<header>
<h1>Understanding Semantic HTML</h1>
<p>Published on July 10, 2024</p>
</header>
<section>
<h2>What is Semantic HTML?</h2>
<p>Semantic HTML uses elements like <code><article></code>, <code><section></code>, and <code><header></code> to describe the content.</p>
</section>
</article>
Custom Data Attributes
Custom data attributes are used to store extra information on HTML elements without using non-standard attributes.
<div data-user-id="12345">User Profile</div>
HTML5 APIs
HTML5 introduces several APIs that allow for more dynamic and interactive web experiences, such as the Geolocation API, Web Storage API, and Canvas API.
<!-- Geolocation API -->
<button onclick="getLocation()">Get My Location</button>
<p id="location"></p>
<script>
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
document.getElementById("location").innerText = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
document.getElementById("location").innerText = "Latitude: " + position.coords.latitude +
" Longitude: " + position.coords.longitude;
}
</script>
Forms and Input Types
HTML5 introduces new input types and form attributes that enhance the functionality and usability of web forms.
<form>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<label for="birthday">Birthday:</label>
<input type="date" id="birthday" name="birthday">
<input type="submit" value="Submit">
</form>
Web Components
Web Components are a set of web platform APIs that allow developers to create custom, reusable HTML elements with encapsulated functionality.
<template id="my-template">
<style>
.card { border: 1px solid #ccc; padding: 10px; border-radius: 5px; }
</style>
<div class="card">
<slot></slot>
</div>
</template>
<script>
class MyCard extends HTMLElement {
constructor() {
super();
const template = document.getElementById('my-template').content;
const shadowRoot = this.attachShadow({ mode: 'open' });
shadowRoot.appendChild(template.cloneNode(true));
}
}
customElements.define('my-card', MyCard);
</script>
<my-card>Content inside my custom card element.</my-card>
Advanced CSS Techniques
CSS Grid Layout
CSS Grid Layout is a two-dimensional layout system for the web. It allows developers to create complex and responsive layouts with ease.
<div class="grid-container">
<div class="grid-item">1</div>
<div class="grid-item">2</div>
<div class="grid-item">3</div>
<div class="grid-item">4</div>
<div class="grid-item">5</div>
<div class="grid-item">6</div>
</div>
<style>
.grid-container {
display: grid;
grid-template-columns: auto auto auto;
gap: 10px;
background-color: #2196F3;
padding: 10px;
}
.grid-item {
background-color: rgba(255, 255, 255, 0.8);
border: 1px solid rgba(0, 0, 0, 0.8);
padding: 20px;
font-size: 30px;
text-align: center;
}
</style>
CSS Flexbox Layout
CSS Flexbox Layout is a one-dimensional layout method for laying out items in rows or columns. It is designed to provide a more efficient way to lay out, align, and distribute space among items in a container.
<div class="flex-container">
<div class="flex-item">1</div>
<div class="flex-item">2</div>
<div class="flex-item">3</div>
</div>
<style>
.flex-container {
display: flex;
justify-content: space-between;
background-color: #f1f1f1;
padding: 10px;
}
.flex-item {
background-color: #4CAF50;
padding: 20px;
font-size: 30px;
text-align: center;
}
</style>
CSS Variables
CSS Variables (Custom Properties) allow you to store values that can be reused throughout a stylesheet, making it easier to manage and maintain your CSS.
<style>
:root {
--primary-color: #4CAF50;
--secondary-color: #ffeb3b;
}
body {
background-color: var(--primary-color);
color: var(--secondary-color);
}
</style>
Advanced Selectors
CSS offers a variety of advanced selectors that allow for more precise targeting of elements.
<style>
/* Attribute Selector */
input[type="text"] {
background-color: yellow;
}
/* Pseudo-class Selector */
a:hover {
color: red;
}
/* Pseudo-element Selector */
p::first-line {
font-weight: bold;
}
/* Child Selector */
ul > li {
list-style: none;
}
/* Adjacent Sibling Selector */
h1 + p {
color: blue;
}
</style>
CSS Transitions and Animations
CSS transitions and animations allow you to add motion to your web pages, enhancing the user experience.
<!-- CSS Transitions -->
<style>
button {
background-color: #4CAF50;
color: white;
padding: 15px 32px;
font-size: 16px;
transition: background-color 0.5s ease;
}
button:hover {
background-color: #45a049;
}
</style>
<!-- CSS Animations -->
<style>
@keyframes example {
from {background-color: red;}
to {background-color: yellow;}
}
div {
width: 100px;
height: 100px;
background-color: red;
animation-name: example;
animation-duration: 4s;
}
</style>
<div></div>
Responsive Design
Responsive design ensures that your website looks good on all devices, regardless of their size. This is achieved using media queries.
<style>
/* Mobile Styles */
body {
background-color: lightblue;
}
@media (min-width: 600px) {
/* Tablet Styles */
body {
background-color: lightgreen;
}
}
@media (min-width: 768px) {
/* Desktop Styles */
body {
background-color: orange;
}
}
</style>
CSS Preprocessors
CSS preprocessors like Sass and LESS add functionality to CSS, making it easier to write and maintain.
// Variables
$primary-color: #4CAF50;
$secondary-color: #ffeb3b;
// Nesting
nav {
ul {
margin: 0;
padding: 0;
list-style: none;
li { display: inline-block; }
}
}
// Mixins
@mixin border-radius($radius) {
-webkit-border-radius: $radius;
-moz-border-radius: $radius;
-o-border-radius: $radius;
border-radius: $radius;
}
.box { @include border-radius(10px); }
CSS Frameworks
CSS frameworks like Bootstrap, Foundation, and Tailwind CSS provide pre-written CSS to speed up development and ensure consistency.
<!-- Bootstrap Example -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<div class="container">
<h1 class="text-center">Hello, world!</h1>
<button class="btn btn-primary">Click me</button>
</div>
Advanced HTML and CSS Project
Project Overview
In this section, we will create a responsive portfolio website
using advanced HTML and CSS techniques discussed above.
Setting Up the Project
- Create a new folder for the project.
- Create the necessary files:
index.html
,style.css
, andscript.js
.
Building the HTML Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Portfolio</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<header>
<nav>
<ul>
<li><a href="#about">About</a></li>
<li><a href="#projects">Projects</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
<main>
<section id="about">
<h1>About Me</h1>
<p>This is the about section.</p>
</section>
<section id="projects">
<h1>Projects</h1>
<p>This is the projects section.</p>
</section>
<section id="contact">
<h1>Contact</h1>
<p>This is the contact section.</p>
</section>
</main>
<footer>
<p>© 2024 My Portfolio</p>
</footer>
</body>
</html>
Styling the Project with CSS
/* Reset some default styles */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
line-height: 1.6;
}
header {
background: #333;
color: #fff;
padding: 1rem 0;
}
header nav ul {
list-style: none;
display: flex;
justify-content: center;
}
header nav ul li {
margin: 0 1rem;
}
header nav ul li a {
color: #fff;
text-decoration: none;
}
main {
padding: 2rem 0;
}
section {
padding: 2rem;
margin-bottom: 2rem;
background: #f4f4f4;
border-radius: 5px;
}
footer {
text-align: center;
padding: 1rem 0;
background: #333;
color: #fff;
}
@media (min-width: 768px) {
main {
display: flex;
flex-direction: column;
align-items: center;
}
section {
width: 80%;
}
}
Adding Interactivity with JavaScript
<script>
document.addEventListener("DOMContentLoaded", function() {
const links = document.querySelectorAll("nav ul li a");
links.forEach(link => {
link.addEventListener("click", function(e) {
e.preventDefault();
const targetId = this.getAttribute("href").substring(1);
document.getElementById(targetId).scrollIntoView({ behavior: "smooth" });
});
});
});
</script>
Conclusion
Mastering advanced HTML and CSS techniques is essential for creating sophisticated, responsive, and user-friendly websites. By understanding and implementing semantic HTML, custom data attributes, HTML5 APIs, CSS Grid, Flexbox, CSS Variables, advanced selectors, transitions, animations, responsive design, and CSS preprocessors, you can significantly enhance your web development skills. Additionally, utilizing CSS frameworks and working on real-world projects like a portfolio website will further solidify your knowledge and prepare you for advanced web development challenges.